TRY2HACK wargame level 4
Introduction
Level 4
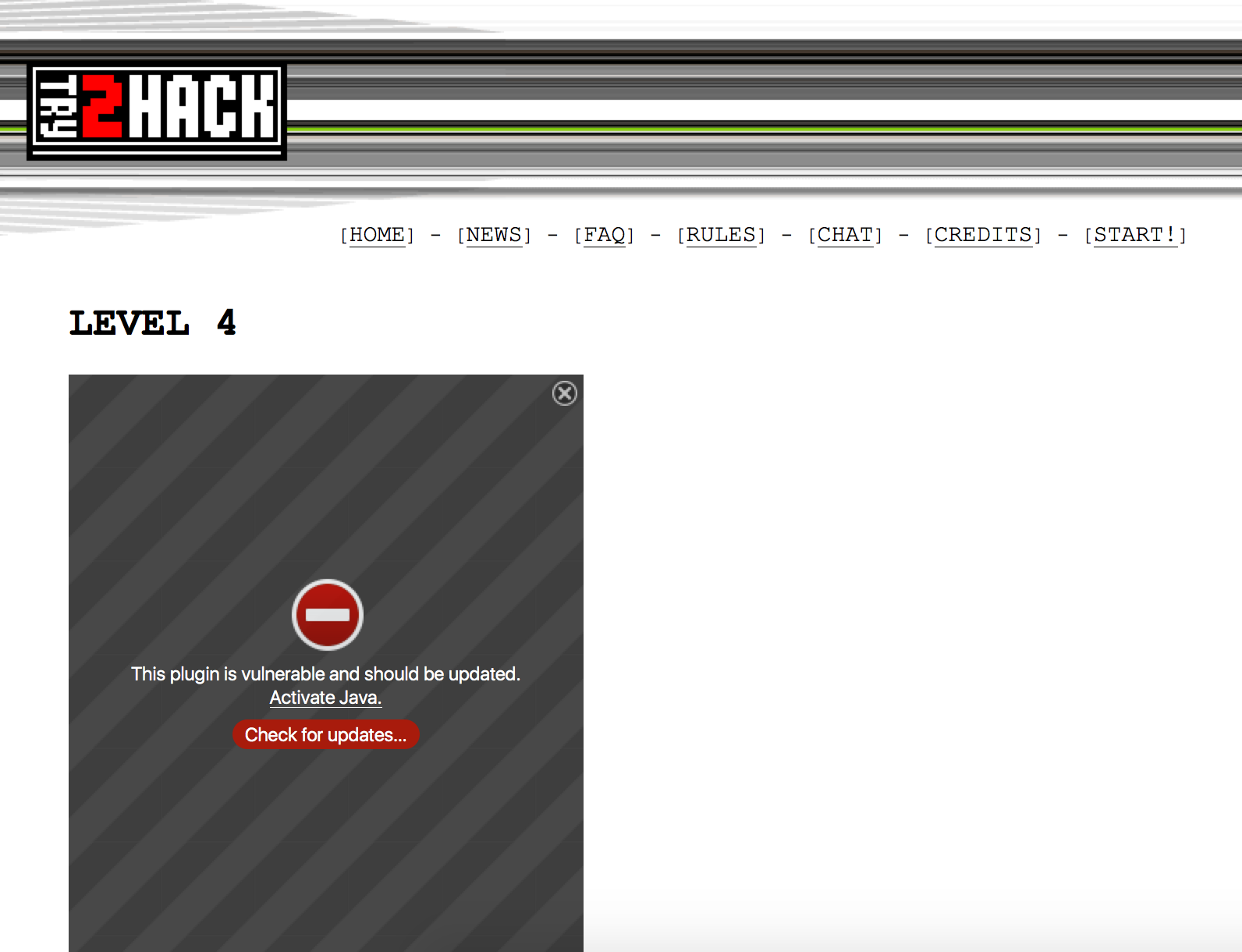
To enable the Java applet, an setting in the system preference is needed.
Choose the Java control panel, choose the security tab, and add the exception site list.
Back to the webpage, click on the activate Java, and choose allow now or allow and remember.
The Java applet is a class object on the webpage. The url pointed to it may be found on the classid attribute. Then we could use applet decompiler to get the source code.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
import java.applet.Applet;
import java.applet.AppletContext;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.*;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.EventObject;
public class PasswdLevel4 extends applet implements actionlistener {
public PasswdLevel4() {
inuser = new string[22];
totno = 0;
countConn = null;
countData = null;
inURL = null;
txtlogin = new textfield();
label1 = new label();
label2 = new label();
label3 = new label();
txtpass = new textfield();
lblstatus = new label();
ButOk = new button();
ButReset = new button();
lbltitle = new label();
}
void ButOk_ActionPerformed(actionevent actionevent) {
boolean flag = false;
for (int i = 1; i <= totno / 2; i++)
if (txtlogin.getText().trim().toUpperCase().intern() == inuser[2 * (i - 1) + 2].trim().toUpperCase().intern() && txtpass.getText().trim().toUpperCase().intern() == inuser[2 * (i - 1) + 3].trim().toUpperCase().intern()) {
lblstatus.setText("Login Success, Loading..");
flag = true;
string s = inuser[1].trim().intern();
string s1 = getParameter("targetframe");
if (s1 == null) s1 = "_self";
try {
finalurl = new url(getCodeBase(), s);
} catch (malformedurlexception _ex) {
lblstatus.setText("Bad URL");
}
getAppletContext().showDocument(finalurl, s1);
}
if (!flag) lblstatus.setText("Invaild Login or Password");
}
void ButReset_ActionPerformed(actionevent actionevent) {
txtlogin.setText("");
txtpass.setText("");
}
public void actionPerformed(actionevent actionevent) {
object obj = actionevent.getSource();
if (obj == ButOk) {
ButOk_ActionPerformed(actionevent);
return;
}
if (obj == ButReset) ButReset_ActionPerformed(actionevent);
}
public void destroy() {
ButOk.setEnabled(false);
ButReset.setEnabled(false);
txtlogin.setVisible(false);
txtpass.setVisible(false);
}
public void inFile() {
new stringbuffer();
try {
countConn = inURL.openStream();
countData = new bufferedreader(new inputstreamreader(countConn));
string s;
while ((s = countData.readLine()) != null)
if (totno < 21) {
totno = totno + 1;
inuser[totno] = s;
s = "";
} else {
lblstatus.setText("Cannot Exceed 10 users, Applet fail start!");
destroy();
}
} catch (ioexception ioexception) {
getAppletContext().showStatus("IO Error:" + ioexception.getMessage());
}
try {
countConn.close();
countData.close();
return;
} catch (ioexception ioexception1) {
getAppletContext().showStatus("IO Error:" + ioexception1.getMessage());
}
}
public void init() {
setLayout(null);
setSize(361, 191);
add(txtlogin);
txtlogin.setBounds(156, 72, 132, 24);
label1.setText("Please Enter Login Name & Password");
label1.setAlignment(1);
add(label1);
label1.setFont(new font("Dialog", 1, 12));
label1.setBounds(41, 36, 280, 24);
label2.setText("Login");
add(label2);
label2.setFont(new font("Dialog", 1, 12));
label2.setBounds(75, 72, 36, 24);
label3.setText("Password");
add(label3);
add(txtpass);
txtpass.setEchoChar('*');
txtpass.setBounds(156, 108, 132, 24);
lblstatus.setAlignment(1);
label3.setFont(new font("Dialog", 1, 12));
label3.setBounds(75, 108, 57, 21);
add(lblstatus);
lblstatus.setFont(new font("Dialog", 1, 12));
lblstatus.setBounds(14, 132, 344, 24);
ButOk.setLabel("OK");
add(ButOk);
ButOk.setFont(new font("Dialog", 1, 12));
ButOk.setBounds(105, 156, 59, 23);
ButReset.setLabel("Reset");
add(ButReset);
ButReset.setFont(new font("Dialog", 1, 12));
ButReset.setBounds(204, 156, 59, 23);
lbltitle.setAlignment(1);
add(lbltitle);
lbltitle.setFont(new font("Dialog", 1, 12));
lbltitle.setBounds(12, 14, 336, 24);
string s = getParameter("title");
lbltitle.setText(s);
ButOk.addActionListener(this);
ButReset.addActionListener(this);
infile = new string("level4");
try {
inURL = new url(getCodeBase(), infile);
} catch (malformedurlexception _ex) {
getAppletContext().showStatus("Bad Counter URL:" + inURL);
}
inFile();
}
private url finalurl;
string infile;
string inuser[];
int totno;
inputstream countConn;
bufferedreader countData;
url inURL;
textfield txtlogin;
label label1;
label label2;
label label3;
textfield txtpass;
label lblstatus;
button ButOk;
button ButReset;
label lbltitle;
}
Input the username and password into the applet and level 5 would come up.
Conclusion
- Class decompiler
- Java applet
- Java Url class
- Java InputStreamReader class
- relative path for a file